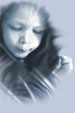 |
 |
|
 |
|
|
|
| Demon:
|
 |
|
|
|
| Dreamcatcher:
|
 |
|
 |
|
 |
|
 |
|
 |
|
|
|
| Shadowlords:
|
 |
|
 |
|
 |
|
 |
|
 |
|
 |
|
|
RemoveEffects
// This is a utility function
// Parameters:
// object oPC: The object you wish to remove an effect from.
// Comments : Note that this will remove only effects created
// by OBJECT_SELF, and it will remove all of them.
void RemoveEffects(object oPC)
{
// Get the first effect (to start looping)
effect ePC = GetFirstEffect(oPC);
// Check that our effect is valid.
// In this case means "while we have more effects"
while (GetIsEffectValid(ePC))
{
// We only want to remove effects that we have placed on the object.
if (GetEffectCreator(ePC) == OBJECT_SELF)
{
RemoveEffect(oPC, ePC);
}
// Get the next effect
ePC = GetNextEffect(oPC);
}
}
Useful script snippets
This applies 100% of the XP for PIRATE_PLOT1 to the PCSpeaker and his party (this one used in dialogue, obviously, as many are).
RewardXP("PIRATE_PLOT1",100,GetPCSpeaker());
This applies 25% of the total XP for HIGHLAND_PLOT2 to the PCSpeaker...but only him, not his party.
RewardXP("HIGHLAND_PLOT2",25,GetPCSpeaker(),FALSE);
void main() // The Mighty Teleport Script, (c) BioWare Corp, 2000
{
object oEnter = GetEnteringObject();
object oDestArea = GetAreaByString("AREA1");
location locEnter = GetLocation(oEnter);
vector vEnter = GetPosition(oEnter)
vector vDest = Vector(vEnter.x, 2.5);
float fFacing = GetFacing(oEnter)
location locNew = Location(oDestArea, vDest, fFacing); // East is at 0.0 radians.
AssignCommand(oEnter, ClearAllActions());
AssignCommand(oEnter, JumpToLocation(locNew));
}
void main()
{
object oPc;
object oItem;
object oMorgrin;
oPc=GetEnteringObject();
oItem=GetItemPossessedBy(oPc,"SilverCirclet");
if(GetIsObjectValid(oItem) == TRUE)
{
location lPlayerlocation;
lPlayerlocation=GetLocation(oPc);
oMorgrin=CreateObject(OBJECT_TYPE_CREATURE, "Morgrin",lPlayerlocation);
AssignCommand(oMorgrin, SpeakString(
"The Ritual is complete. Draw the Circle, call forth the blue flame!"));
}
}
SetLocalInt(OBJECT_SELF, "GivenQuest",1);
GetLocalInt(OBJECT_SELF,"GivenQuest");
DestroyLocalInt(OBJECT_SELF,"GivenQuest");
CreateItemOnObject(?)
void main ()
{
if(GetIsResting(OBJECT_SELF)
{
effect eQASleep = EffectSleep();
ClearAllActions();
RemoveEffect(OBJECT_SELF, eQASleep);
ExecuteScript("NW_C2_GETOUTOFBED");
AssignCommand(OBJECT_SELF,ActionMoveToLocation(GetLocation(GetWaypointByTag("WP_STARBUCKS")),TRUE));
SetLocalInt(OBJECT_SELF,"CAFFINE_INTAKE",20);
AssignCommand(OBJECT_SELF,ActionMoveToLocation(GetLocation(GetWaypointByTag("WP_QA_ASYLUM")),TRUE));
}
}
object oMace = GetItemPossessedBy(PC, "powerful_mace");
if (GetIsObjectValid(oMace))
do whatever...
object oPC = GetEnteringObject();
int iNatureLevel = GetLevelByClass(CLASS_TYPE_DRUID, oPC) + GetLevelByClass(CLASS_TYPE_RANGER, oPC);
if (iNatureLevel > 0)
{
int iWisdom = GetAbilityModifier(ABILITY_WISDOM, oPC);
if ((d20() + iWisdom) > 12)
{
// there may be a way to direct this line directly to the
// druid via TALKVOLUME_WHISPER... I'm just not sure how, myself
SpeakString("The rabbits seem to be very odd in their behavior...");
}
}
// Close door 10 seconds after being opened
void main(){
DelayCommand(10.0, ActionCloseDoor(OBJECT_SELF));
}
void GiveGoldToCreature(object oCreature, int nGP)
void GiveXPToCreature( object oCreature, int nXpAmount )
int GetJournalQuestExperience(string szPlotID)
//How much gold does someone have?
int GetGold( object oTarget = OBJECT_SELF )
//if you want someone to take gold from someone else, you use this:
void TakeGoldFromCreature( int nAmount, object oCreatureToTakeFrom, int bDestroy = FALSE )
If 'bDestroy' is marked as TRUE, then the creature taking the gold doesn't end up with it in their inventory
the gold is destroyed and removed from the world.
//To give 100 XP to each member of the party, starting with the nearest one to me:
object oFirstMember = GetNearestCreature(CREATURE_TYPE_PLAYER_CHAR, PLAYER_CHAR_IS_PC);
object oPartyMember = GetFirstFactionMember(oFirstMember, TRUE);
while (GetIsObjectValid(oPartyMember) == TRUE)
{
GiveXPToCreature(oPartyMember,100);
oPartyMember = GetNextFactionMember(oFirstMember, TRUE);
}
}
//Official campaign function:
int AutoDC(int DC, int nSkill, object oTarget)
//So if I wanted to make a persuade of an NPC that the PC is in dialogue with a hard test, I could use this:
AutoDC(DC_HARD, SKILL_PERSUADE, GetPCSpeaker());
//This returns the rank + ability bonus of the oTarget's skill.
int GetSkillRank(int nSkill, object oTarget=OBJECT_SELF)
//This returns the creature's level in a particular class (such as CLASS_TYPE_FIGHTER).
int GetLevelByClass(int nClassType, object oCreature = OBJECT_SELF)
//This is useful if you want the character level of the PC, regardless of their class(es).
int GetHitDice(object oCreature)
//Gets the modifier for a particular ability, useful if you want a strength check only, or something similar.
int GetAbilityModifier(int nAbility, object oCreature=OBJECT_SELF)
//Damage a player:
effect EffectDamage(int nDamage, int nDamageType=DAMAGE_TYPE_MAGICAL, int nDamagePower=DAMAGE_POWER_NORMAL)
ApplyEffectToObject(DURATION_TYPE_INSTANT, EffectDamage(1), oPC);
// first remove the clothing you're wearing
object oClothing = GetItemInSlot(INVENTORY_SLOT_CHEST);
if (GetIsObjectValid(oClothing))
{
ActionUnequipItem(oClothing);
// might want to destroy it or put it somewhere, but for now drop it
ActionPutDownItem(oClothing);
}
// now determine which of the 6 clothing items is to be worn
int iRandom = d6();
string sChange = "CLOTHING" + IntToString(iRandom);
// create it in inventory and put it on
ActionDoCommand(CreateItemOnObject(sChange));
ActionEquipItem(GetItemPossessedBy(OBJECT_SELF,sChange), INVENTORY_SLOT_CHEST);
//Begin a conversation
BeginConversation("dialoguefilename",GetPCSpeaker());
SpeakString just makes a string appear over the head. It has nothing to do with the dialogue file.
SpeakOneLinerConversation does initiate dialogue... but it is designed to have the
NPC starting their own dialogue file without setting off their OnDialogue event.
They say one line, no responses... so it has the same effect, really, as SpeakString.
The difference? You can have scripts sorting through what lines to speak and you can use tokens (him/her, he/she, etc.)
ActionStartConversation is the command you would normally want to initiate a full dialogue file.
//To spawn in a wizard with the creation effect, facing the PC...
object oWay = GetWaypointByTag("WAYPOINT1");
vector vPos = GetPosition(oWay);
vector vPC = GetNearestCreatureToLocation(CREATURE_TYPE_PLAYER_CHAR, PLAYER_CHAR_IS_PC, vPos);
location lPos = Location(GetArea(oWay), vPos, SetFacingPoint(vPC));
CreateObject(OBJECT_TYPE_CREATURE, "wizard01", lPos, TRUE);
// Run to a waypoint
ActionMoveToObject(oWaypoint, TRUE);
object GetNearestCreature(int nFirstCreatureType, int nFirstParameter, object oTarget=OBJECT_SELF, int nNth=1, int nSecondCreatureType = -1, int nSecondParameter = -1, int nThirdCreatureType = -1, int nThirdParameter = -1 )
//The nearest enemy wizard?
GetNearestCreature(CREATURE_TYPE_REPUTATION, REPUTATION_TYPE_ENEMY, OBJECT_SELF, 1, CREATURE_TYPE_CLASS, CLASS_TYPE_WIZARD);
//The nearest living enemy to my nearest PC ally?
GetNearestCreature(CREATURE_TYPE_REPUTATION, REPUTATION_TYPE_ENEMY, (GetNearestCreature(CREATURE_TYPE_PLAYER_CHAR, PLAYER_CHAR_IS_PC, OBJECT_SELF, 1, CREATURE_TYPE_REPUTATION, REPUTATION_TYPE_FRIENDLY), 1, CREATURE_TYPE_IS_ALIVE, TRUE);
//The nearest non-PC that I can see?
GetNearestCreature(CREATURE_TYPE_PLAYER_CHAR, PLAYER_CHAR_NOT_PC, OBJECT_SELF, 1, CREATURE_TYPE_PERCEPTION, PERCEPTION_TYPE_SEEN);
object GetNearestObject(int nObjectType=OBJECT_TYPE_ALL, object oTarget=OBJECT_SELF, int nNth=1)
//'nNth' is the integer that allows you to go beyond the 'nearest'... an nNth of 3 would look for the 'third nearest', for example.
OBJECT_TYPE_ALL, OBJECT_TYPE_AREA_OF_EFFECT, OBJECT_TYPE_CREATURE, OBJECT_TYPE_DOOR, OBJECT_TYPE_INVALID, OBJECT_TYPE_ITEM
OBJECT_TYPE_PLACEABLE, OBJECT_TYPE_STORE, OBJECT_TYPE_TRIGGER, OBJECT_TYPE_WAYPOINT
float GetDistanceBetween(object oObjectA, object oObjectB)
//For finding distance between self and an object
float GetDistanceToObject(object oObject)
//Unique item properties
//OnAcquireItem event
void main ()
{
object oItem = GetObjectByTag("SWORD01");
int iVar = GetLocalInt(GetItemPossessor(oItem), "VARIABLENAME");
// below is 'if the firer of the event is the one I've specified
// and the variable hasn't been set, set the variable on the
// item's possessor'
if ((GetModuleItemAcquired() == oItem) && (iVar == 0))
{
SetLocalInt(GetItemPossessor(oItem), "VARIABLENAME", 1);
}
}
//There is also an OnUnAcquireItem event and an GetModuleItemUnAcquired() function
//This returns the item that caused the last OnActivateItem() event.
object GetItemActivated()
//This returns the target of the Unique Power, if it was targeted at a specific object.
object GetItemActivatedTarget()
//This returns the target of the Unique Power, if it was targeted at a location rather than a specific object.
location GetItemActivatedTargetLocation()
//This returns the creature object of whoever activated the item... a bit handier than going through the whole GetItemPossessedBy rigamarole.
object GetItemActivator()
// Returns the successful attack target of the specified creature. This
// function only works when the target creature is in combat.
object GetAttackTarget(object oTarget=OBJECT_SELF)
// Returns the target that you attempted to attack. Should probably
// be used in conjunction with GetAttackTarget() This function is
// set every time you attempt to attack a creature.
object GetAttemptedAttackTarget()
// This function is set every time you attempt to cast a spell at
// a creature and is reset at the end of combat.
object GetAttemptedSpellTarget()
// Get oTarget's challenge rating.
// This will only work for creatures.
// A 0.0 is returned if the target is invalid.
float GetChallengeRating( object oTarget )
// Check whether the creature has the specified talent (i.e. spell, skill, feat or combat mode)
int GetCreatureHasTalent(talent tTalent, object oCreature=OBJECT_SELF)
// Get the best talent of the creature of the specified CR, within the specified category
talent GetCreatureTalentBest(int nCategory, int nCRMax, object oCreature=OBJECT_SELF)
// Get a random talent of the creature, within the specified category
talent GetCreatureTalentRandom(int nCategory, object oCreature=OBJECT_SELF)
Plus a whole bunch of faction-related gets along this line:
// Returns the object id of the member of the specified faction that has taken the most hit points of damage
object GetFactionMostDamagedMember(object oFactionMember=OBJECT_SELF, int bMustBeVisible=TRUE)
// Returns TRUE if the Target considers the Source as an enemy.
int GetIsEnemy(object oTarget, object oSource=OBJECT_SELF)
// Functions for Combat. Combat is good.
object GetLastAttacker(object oAttackee=OBJECT_SELF)
// Returns the last object that damaged this object
object GetLastDamager()
// Returns the successful attack target of the specified creature. This
// function only works when the target creature is in combat.
object GetAttackTarget(object oTarget=OBJECT_SELF)
// Returns the target that you attempted to attack. Should probably
// be used in conjunction with GetAttackTarget() This function is
// set every time you attempt to attack a creature.
object GetAttemptedAttackTarget()
// This function is set every time you attempt to cast a spell at
// a creature and is reset at the end of combat.
object GetAttemptedSpellTarget()
// Get oTarget's challenge rating.
// This will only work for creatures.
// A 0.0 is returned if the target is invalid.
float GetChallengeRating( object oTarget )
// Check whether the creature has the specified talent (i.e. spell, skill, feat or combat mode)
int GetCreatureHasTalent(talent tTalent, object oCreature=OBJECT_SELF)
// Get the best talent of the creature of the specified CR, within the specified category
talent GetCreatureTalentBest(int nCategory, int nCRMax, object oCreature=OBJECT_SELF)
// Get a random talent of the creature, within the specified category
talent GetCreatureTalentRandom(int nCategory, object oCreature=OBJECT_SELF)
Plus a whole bunch of faction-related gets along this line:
// Returns the object id of the member of the specified faction that has taken the most hit points of damage
object GetFactionMostDamagedMember(object oFactionMember=OBJECT_SELF, int bMustBeVisible=TRUE)
// Returns TRUE if the Target considers the Source as an enemy.
int GetIsEnemy(object oTarget, object oSource=OBJECT_SELF)
// Functions for Combat. Combat is good.
object GetLastAttacker(object oAttackee=OBJECT_SELF)
// Returns the last object that damaged this object
object GetLastDamager()
//There are three constants for talent types:
TALENT_TYPE_FEAT
TALENT_TYPE_SKILL
TALENT_TYPE_SPELL
//Breaking it down, there are numerous talent categories that spells, feats and skills can be labeled with:
int TALENT_CATEGORY_BENEFICIAL_CONDITIONAL_AREAEFFECT
int TALENT_CATEGORY_BENEFICIAL_CONDITIONAL_POTION
int TALENT_CATEGORY_BENEFICIAL_CONDITIONAL_SINGLE
int TALENT_CATEGORY_BENEFICIAL_ENHANCEMENT_AREAEFFECT
int TALENT_CATEGORY_BENEFICIAL_ENHANCEMENT_POTION
int TALENT_CATEGORY_BENEFICIAL_ENHANCEMENT_SELF
int TALENT_CATEGORY_BENEFICIAL_ENHANCEMENT_SINGLE
int TALENT_CATEGORY_BENEFICIAL_HEALING_AREAEFFECT
int TALENT_CATEGORY_BENEFICIAL_HEALING_POTION
int TALENT_CATEGORY_BENEFICIAL_HEALING_TOUCH
int TALENT_CATEGORY_BENEFICIAL_OBTAIN_ALLIES
int TALENT_CATEGORY_BENEFICIAL_PROTECTION_AREAEFFECT
int TALENT_CATEGORY_BENEFICIAL_PROTECTION_POTION
int TALENT_CATEGORY_BENEFICIAL_PROTECTION_SELF
int TALENT_CATEGORY_BENEFICIAL_PROTECTION_SINGLE
int TALENT_CATEGORY_DRAGONS_BREATH
int TALENT_CATEGORY_HARMFUL_AREAEFFECT_DISCRIMINANT
int TALENT_CATEGORY_HARMFUL_AREAEFFECT_INDISCRIMINANT
int TALENT_CATEGORY_HARMFUL_RANGED
int TALENT_CATEGORY_HARMFUL_TOUCH
int TALENT_CATEGORY_PERSISTENT_AREA_OF_EFFECT
// Finding the type of an item
GetBaseItemType(object oItem)
//Returns: BASE_ITEM_GOLD, BASE_ITEM_KATANA, BASE_ITEM_KEY, BASE_ITEM_MISCSMALL, etc.
//These are custom global tokens that you can set in scripts. The grammar is:
void SetCustomToken(int nCustomTokenNumber, string sTokenValue)
//So if I use SetCustomToken(100, GetName(GetObjectByTag("SWORD002"))) I could then, in dialogue, use:
"Would you like to take a look at this fine ?"
//GetFirstItemInInventory and then a while-loop with GetNextItemInInventory
oItem = GetFirstItemInInventory(oPC)
while (GetIsObjectValid(oItem) == TRUE)
{
oItem = GetNextItemInInventory(oPC)}
}
// Who last opened a door
GetLastOpenedBy()
// Who last used an item
GetLastUsedBy()
// OnInventoryDisturbed events:
You would put a script in the OnInventoryDisturbed() section of the container:
Check to see if GetInventoryDisturbType() returns as INVENTORY_DISTURB_TYPE_ADDED.
If so, use GetInventoryDisturbItem() to get the item added and send it back to the returned object of GetLastDisturbed().
//OnPlayerRest event
OnPlayerRest event is a tri-state event... it will fire once when the player begins rest...
once when it is cancelled and again when the rest is complete...
// follow this object until a ClearAllActions is called.
void ActionForceFollowObject(object oFollow, float fFollowDistance=0.0f)
// Effects
effect eConPenalty = EffectAbilityDecrease(ABILITY_CONSTITUTION, 1);
ApplyEffectToObject(DURATION_TYPE_PERMANENT, eConPenalty, oTargetPC);
// Death and dying
OnPlayerDeath(), OnPlayerDying() and OnPlayerRespawn().
GetLastPlayerDying()
//This returns the last PC who fired the module's OnPlayerDying() event (when the player is at 0 HP and supposed to be dead...
the standard script applies EffectDeath() on him so he goes ahead and just dies).
GetLastPlayerDied()
//This returns the last PC who fired the module's OnPlayerDeath() event. This means a player is actually fully dead...
the standard script determines the effect of death in certain special places within the campaign and, otherwise, calls the PopUpGUIPanel command to allow the player to decide what to do (respawn, wait for help, load, quit).
GetLastRespawnButtonPresser()
//This returns the last player who pressed the respawn button on that pop-up GUI panel.
The standard script has him resurrected in the nearest temple within the campaign module,
heals him and scans him for any negative effects (and removes them).
effect GetFirstEffect(object oCreature)
effect GetNextEffect(object oCreature)
object GetFirstFactionMember(object oMemberOfFaction, int bPCOnly = TRUE)
object GetNextFactionMember(object oMemberOfFaction, int bPCOnly = TRUE)
// Get the first object within the specified persistent object.
// nResidentObjectType is the type of object we want, nPersistentZone indicates whether
// to look in the active area, or if the PersistentObject is an Encounter, in the follow area.
object GetFirstInPersistentObject(object oPersistentObject = OBJECT_SELF, int nResidentObjectType = OBJECT_TYPE_CREATURE, int nPersistentZone = PERSISTENT_ZONE_ACTIVE)
object GetNextInPersistentObject(object oPersistentObject = OBJECT_SELF, int nResidentObjectType = OBJECT_TYPE_CREATURE, int nPersistentZone = PERSISTENT_ZONE_ACTIVE)
(don't ask me about the one above)
object GetFirstItemInInventory(object oTarget = OBJECT_SELF)
object GetNextItemInInventory(object oTarget = OBJECT_SELF)
// if no valid area specified, then use the area that the caller is in.
object GetFirstObjectInArea(object oArea = OBJECT_INVALID)
object GetNextObjectInArea(object oArea = OBJECT_INVALID)
// this is generally used for determining which creatures
// lie in the radius of a spell or effect
object GetFirstObjectInShape(int nShape, float fSize, location lTarget, int bLineOfSight=FALSE, int nObjectFilter=OBJECT_TYPE_CREATURE, vector vOrigin=[0.0,0.0,0.0])
object GetNextObjectInShape(int nShape, float fSize, location lTarget, int bLineOfSight=FALSE, int nObjectFilter=OBJECT_TYPE_CREATURE, vector vOrigin=[0.0,0.0,0.0])
// Returns the object of the first PC in the player list. This resets where in the player
// list GetNextPC() retrives PC objects from.
object GetFirstPC()
// Returns the object of the next PC in the player list. This picks up where the last
// GetFirstPC() or GetNextPC() call left off.
object GetNextPC()
Well, it depends on what you plan on using it for. Let's say you had an NPC who is willing to give the PC something in exchange for a magic ring of at least 1000 gp value. The dialogue starts as normal. The NPC has the following line somewhere after the deal is offered: "So then, ... do you have a ring that you're willing to trade me?" So, then... first thing I do is in the action script of the NPC line above:
void main(){ // find out how many rings the PC has and mark them int iRingNum = 0; object oItem = GetFirstItemInInventory(GetPCSpeaker()); while (GetIsObjectValid(oItem)) { if (GetBaseItemType(oItem) == OBJECT_TYPE_RING) { iRingNum++; SetCustomToken((iRingNum + 1000), GetName(oItem)); SetLocalObject(OBJECT_SELF, "RING" + IntToString(iRingNum), oItem); } oItem = GetNextItemInInventory(GetPCSpeaker()); } // hold onto that number of rings SetLocalInt(OBJECT_SELF, "NumberRings", iRingNum);}
(note: the 'iRingNum++' and the inside brace just won't tab right no matter what I do. Sorry.)
I then have to list player replies linked to that NPC line... and each needs a 'starting condition' script that ends up being true or false, right? So I write the following replies:
A. I don't have any rings right now.
B. How about this ?
C. How about this ?
D. How about this ?
E. Keep looking for more rings.
F. Forget it.
Ok, then... script for line A is simple:
int StartingConditional()
{
int iRingNum;
iRingNum = GetLocalInt(OBJECT_SELF, "NumberRings") == 0;
return iRingNum;
}
So if "NumberRings" is equal to 0, the script returns TRUE... and that means player response A will appear. Script for line B:
int StartingConditional(){ int iRingNum; iRingNum = GetLocalInt(OBJECT_SELF, "NumberRings") > 0; return iRingNum;}
So if there's at least 1 ring, line B appears... and the custom token for 1001 will already have been set. Similar scripts for C, D and E can be set... C looking for "NumberRings" to be > 1 and so on.
So if B, C or D is selected, we already know which exact item in inventory we are talking about, right? If E is selected, I can insert a blank node and list more rings (depending on how tight I want to keep the on-screen list).
So let's say that the player selects B. I run an action script on the B response line that grabs the object from "RING1" and assigns it to another object integer called "CHOSENRING"... and then I could branch to three separate lines of dialogue:
branch 1: "Hmm... that worth far more than I'm asking. Are you sure you want to part with it?"
branch 2: "That's a mighty fine ring, all right... easily worth what I'm asking. You have a deal."
branch 3: "No, that ring's not worth enough."
I would assign the following script to the starting condition for branch one:
int StartingConditional(){ object oRing = GetLocalObject(OBJECT_SELF, "CHOSENRING"); if (GetGoldPieceValue(oRing) > 2000) { return TRUE; } return FALSE;}
So if the ring was worth more than 2000 gp, branch 1 is TRUE and is followed. I could put this script in branch 2 but look for a value of < 999 (meaning the value is 1000-2000 gp)... if it returns TRUE, branch 2 is followed. No script is needed for branch 3... no script on a branch means it is the default and automatically TRUE. If the branch is even reached, the value of the ring is < 1000, right? Does that make more sense, then, how the scanning might be used?
MODULE EVENTS
Event: OnAcquireItem, used in: Module, what triggered it? Use object GetModuleItemAcquired(). Also used here: object GetModuleItemAcquiredFrom(), object GetItemPossessor(object oItem)
Event: OnActivateItem, used in: Module, what triggered it? Use object GetItemActivated(). Also used here: object GetItemActivatedTarget(), location GetItemActivatedTargetLocation(), object GetItemActivator()
Event: OnClientEnter, used in: Module, what triggered it? Use GetEnteringObject()
Event: OnClientLeave, used in: Module, what triggered it? Use GetExitingObject()
Event: OnModuleLoad, used in: Module, what triggered it? The module itself... there is no specific object to 'get' in this case. In effect, this is the module version of the OnSpawn script, run only once when the module is loaded.
Event: OnPlayerDeath, used in: Module, what triggered it? Use object GetLastPlayerDied(). Also used here: object GetLastKiller()
Event: OnPlayerDying, used in: Module, what triggered it? Use object GetLastPlayerDying(). The difference between this and OnPlayerDeath is that here the PC is out of HP but hasn't officially 'died' yet. The standard script here is to apply EffectDeath to the player and officially kill him, but the option is open for you.
Event: OnPlayerLevelUp, used in: Module, what triggered it? Use object GetPCLevellingUp(). This could be used to prevent someone from leveling up (except, say, until trained by an NPC) or detect when someone has leveled up... it is not used to control the level-up process.
Event: OnPlayerRespawn, used in: Module, what triggered it? Use object GetLastRespawnButtonPresser(). Here you would indicate where a player was respawned and what would occur upon respawning (such as a Resurrection).
Event: OnPlayerRest, used in: Module, what triggered it? Use object GetLastPCRested(). Also used here: int GetLastRestEventType() --- note that this event fires both before and after a PC rest. You can detect which through the use of GetLastRestEventType(), with the constants REST_EVENTTYPE_REST_CANCELLED, REST_EVENTTYPE_REST_FINISHED, REST_EVENTTYPE_REST_INVALID, REST_EVENTTYPE_REST_STARTED.
Event: OnUnAcquireItem, used in: Module, what triggered it? Use object GetModuleItemLost(). Also used here: object GetModuleItemLostBy()
AREA EVENTS
Event: OnEnter, used in: Area, what triggered it? Use object GetEnteringObject().
Event: OnExit, used in: Area, what triggered it? Use object GetExitingObject().
CREATURE EVENTS
Event: OnPerception, used in: Creature, what triggered it? Use object GetLastPerceived(). Also used here: int GetLastPerceptionHeard(), int GetLastPerceptionInaudible(), int GetLastPerceptionSeen(), int GetLastPerceptionVanished()
Event: OnSpellCastAt, used in: Creature, what triggered it? Use object GetLastSpell(). Also used here: object GetLastSpellCaster(), int GetLastSpellHarmful()
Event: OnPhysicalAttacked, used in: Creature, what triggered it? Use object GetLastAttacker(). Also used here: object GetLastDamager()
Event: OnDamaged, used in: Creature, what triggered it? Use object GetLastDamager(). Also used here: int GetTotalDamageDealt(), int GetDamageDealtByType(int nDamageType), int GetCurrentHitPoints(object oObject = OBJECT_SELF), int GetMaxHitPoints(object oObject = OBJECT_SELF)
Event: OnDisturbed, used in: Creature, what triggered it? Use object GetLastDisturbed(). Also used here: int GetInventoryDisturbType() = int INVENTORY_DISTURB_TYPE_ADDED, int INVENTORY_DISTURB_TYPE_REMOVED, int INVENTORY_DISTURB_TYPE_STOLEN, object GetInventoryDisturbItem()
Event: OnCombatRoundEnd, used in: Creature, what triggered it? Fires when creature has been in combat for one full round, at the end of each combat round.
Event: OnConversation, used in: Creature, what triggered it? Use object GetLastSpeaker(). Also used here: int GetListenPatternNumber()
Event: OnRested, used in: Creature, what triggered it? Fires when the NPC rests via ActionRest().
Event: OnDeath, used in: Creature, what triggered it? Fires when the NPC is killed.
Event: OnBlocked, used in: Creature, what triggered it? Use object GetBlockingDoor(). Note that only doors currently trigger the OnBlocked event... this is not used for collision detection (yet).
Event: OnClose , used in: Placeable, what triggered it? The object has been closed. Note that not all placeable objects can be 'opened' and 'closed' (containers, mostly). Those that can't should use the OnUsed event, instead, for interactions.
Event: OnDamaged, used in: Placeable, what triggered it? Use object GetLastDamager().
Event: OnDeath, used in: Placeable, what triggered it? the object has been destroyed (playing its 'destruction' animation is automatic). object GetLastKiller() will return the object that destroyed the placeable.
Event: OnDisturbed, used in: Placeable, what triggered it? Use int GetInventoryDisturbType() . This results in one of these constants: INVENTORY_DISTURB_TYPE_ADDED, INVENTORY_DISTURB_TYPE_REMOVED, INVENTORY_DISTURB_TYPE_STOLEN. Also used here: object GetLastDisturbed(), object GetInventoryDisturbItem()
Event: OnLock, used in: Placeable, what triggered it? Use object GetLastLocked() . Any placeable object that is marked as a container (having an inventory) can also be marked as 'relockable' (you can even make a key required or give it a DC to re-lock).
Event: OnPhysicalAttacked, used in: Placeable, what triggered it? Use object GetLastAttacker().
Event: OnOpen, used in: Placeable, what triggered it? Use object GetLastOpenedBy().
Event: OnSpellCastAt, used in: Placeable, what triggered it? Use object GetLastSpellCaster(). Also used here: int GetLastSpell()
Event: OnUnlock, used in: Placeable, what triggered it? Use object GetLastUnlocked().
Event: OnUsed, used in: Placeable, what triggered it? Use object GetLastUsedBy() . Any placeable object can be marked as 'useable', which means this script will be activated when it is clicked upon. I believe (though I am not sure) that if there is no OnUsed script and the object is a container, it would subsequently open (or attempt to open) the container after a click.
ActivatePortal(oPlayer, "192.168.0.25:8000", "secretpassword", "wp_arrival", TRUE)
You call the above when the client hits the trigger/door/use object/whatever with oPlayer being the player object,
"192.168.0.25:8000" being the target IP:port of the server to portal to,
"secretpassword" being the Player password for the target server,
"wp_arrival" being the target Waypoint's tag,
and TRUE meaning to make it a seemless transition where the client doesn't see the password dialog.
If you set it to FALSE, then they are prompted for the Player password for the target server.
// OnPlayerRest event -- Can only rest every 8 hours
void main()
{
if (GetLastRestEventType() == REST_EVENTTYPE_REST_STARTED)
{
object oPC = GetLastPCRested();
int nLastRest = GetLocalInt(oPC, "LastRest");
int nHour = GetTimeHour();
// check to see if the last rest was late in the day
if ((nLastRest > 16) && ((nLastRest - nHour) < 16))
{
AssignCommand(oPC, ClearAllActions());
}
// if not, make sure at least 8 hours has passed
else if ((nLastRest < 17) && ((nHour - nLastRest) < 8) )
{
AssignCommand(oPC, ClearAllActions());
}
else SetLocalInt(oPC, "LastRest", nHour);
}
}
//finds out whether oNPC is a friend of the creature running the script.
GetIsFriend(oNPC, OBJECT_SELF)
// Change alignment
void AdjustAlignment(object oSubject, int nAlignment, int nShift)
nAlignment is the constant... which are as follows:
ALIGNMENT_ALL
ALIGNMENT_GOOD
ALIGNMENT_EVIL
ALIGNMENT_LAWFUL
ALIGNMENT_CHAOTIC
The nShift is the number value that the alignment is shifted towards the constant used.
If you just want to use straight positive and negative numbers, you can use ALIGNMENT_ALL.
Otherwise the number will automatically gravitate them towards the alignement specified (without positive or negative numbers being needed).
void ApplyEffectToObject(int nDurationType, effect e, object oTarget, float fDuration = 0.0f)
//With this effect:
effect EffectSkillIncrease(int nSkill, int nIncreaseBy)
//So to increase your merchant's skill by 1:
object oMerchant = GetObjectByTag("MERCHANT");
effect eSkill = EffectSkillIncrease(SKILL_SPOT, 1);
ApplyEffectToObject(DURATION_TYPE_PERMANENT, eSkill, oMerchant);
// Dialog tokens
, and will return the appropriate tokens of the target of a dialogue automatically.
*
*
*
* (neutral possible)
*
*
*
*
*
* (neutral possible)
* (neutral possible)
*
*
*
*
*
* (results in adjective, such as 'elven')
*
Those I marked with an * beside them have a no-caps version, as well...
if you want 'sir' instead of 'Sir', you simply write the tag without capitals =
Action = green
Check = red
Highlight = blue
You just need to use , or before the text you want
colored and then directly after it.
current character level = int GetHitDice(object oCreature)
current level in a class = int GetLevelByClass(int nClassType, object oCreature = OBJECT_SELF)
experience points = int GetXP(object oCreature)
see if a chair is occupied by someone else (via GetSittingObject)
// Put random amount of gold in chest - add to OnOpen & OnDestroyed
void main()
{
int iRandom = d100();
CreateItemOnObject("NW_IT_GOLD001",OBJECT_SELF,iRandom);
}
// heal player
void main()
{
ActionPauseConversation();
ActionCastSpellAtObject(SPELL_HEAL, GetPCSpeaker());
ActionWait(1.0);
ActionResumeConversation();
}
// secret door stuff . . .
void main()
{
int searchRoll;
int found;
object door;
found = GetLocalInt(GetArea(), "found");
door = GetObjectByTag("SecretDoor");
if (found != 1)
{
searchRoll = d20() + GetSkillRank(SKILL_SEARCH, GetLastUsedBy());
if (searchRoll >= 15)
{
ActionSpeakString("This wall looks weathered.",TALKVOLUME_TALK);
ActionStartConversation(GetLastUsedBy(), "", TRUE);
SetLocalInt(GetArea(OBJECT_SELF), "found", 1);
}
}
}
void main()
{
object oGem = GetObjectByTag("GEM_01");
ActionTakeItem(oGem, GetPCSpeaker());
// the following gives 100 gp
GiveGoldToCreature(GetPCSpeaker(), 100);
// OR the following gives 100 XP
GiveXPToCreature(GetPCSpeaker(), 100);
// and let's have them leave the area now that they're done
ActionMoveAwayFromObject(GetPCSpeaker());
DestroySelf(OBJECT_SELF, 10.0);
SetCommandable(FALSE);
}
// Attach to trigger - speak when entered
main void()
{
int iTimes = GetLocalInt(OBJECT_SELF, "Do_once");
if (iTimes == 0)
{
SetLocalInt(OBJECT_SELF, "Do_once", 1);
ActionSpeakString("You have entered a place that seems...");
}
}
So here's a script that I put on my lamp post's OnUsed event. If the lamp post is on, it turns it off. If the lamp post is off, it turns it on.
void main()
{
// Get the current illumination state
int nActivated = GetPlaceableIllumination(OBJECT_SELF);
// Is the light on?
if (nActivated)
{
// the light is on, so turn it off
PlayAnimation(ANIMATION_PLACEABLE_DEACTIVATE);
SetPlaceableIllumination(OBJECT_SELF, FALSE);
}
else
{
// the light is off, so turn it on
PlayAnimation(ANIMATION_PLACEABLE_ACTIVATE);
SetPlaceableIllumination(OBJECT_SELF, TRUE);
}
// this function MUST be called for the players to see the lighting changes RecomputeStaticLighting(GetArea(OBJECT_SELF));}
If you want to see the switch activate place this line in the onUsed event script:
PlayAnimation(ANIMATION_PLACEABLE_ACTIVATE);
//Random waypoints
string sWaypoint;
int i;
sWaypoint = IntToString(d20());
i = GetStringLength(sWaypoint);
if (i == 1)
{
sWaypoint = IntToString(0) + sWaypoint;
}
sWaypoint = "wpnt" + sWaypoint;
Code:
// oObject = object on which to save the value
// sVarName = name of the array
// nIndex = well, the index
// nValue = the value of coursevoid
SetIntArray(object oObject, string sVarName, int nIndex, int nValue)
{
SetLocalInt(oObject, sVarName+IntToString(nIndex), nValue);
return;
}
int GetIntArray(object oObject, string sVarName, int nIndex)
{
return GetLocalInt(oObject, sVarName+IntToString(nIndex));
}
//examples
//set the 4th position of array "penguin" to 42, store it on OBJECT_SELF:
SetIntArray(OBJECT_SELF, "penguin", 4, 42);
// retrieve the value at the 894th position of array "deflator_mouse"
int nFred = GetIntArray(OBJECT_SELF, "deflator_mouse", 894);
void main()
{
object oItemY = GetObjectByTag("OBJECT_Y");
if (GetModuleItemAcquired() == oItem)
{
AddJournalQuestEntry("quest tag here", 2, GetItemPossessor(oItemY));
}
}
// Send message to a player when they enter an area.
void main()
{
object oPlayer;
oPlayer = GetEnteringObject();
SendMessageToPC(oPlayer,"Hey, how's it going?");
}
//Assign the conversation (consisting of one line) to the object. Then make this the object's OnUsed script:
//Make sure the 'Useable' flag is set in the Placeable Properties Editor.
void main()
{
ActionStartConversation(OBJECT_SELF);
}
// Healing fountain
void main()
{
object oUser;
effect eHeal;
int nCurrentHitPoints, nMaxHitPoints;
oUser = GetLastUsedBy();
nMaxHitPoints = GetMaxHitPoints(oUser);
nCurrentHitPoints = GetCurrentHitPoints(oUser);
if ( nCurrentHitPoints < nMaxHitPoints )
{
eHeal = EffectHeal(nMaxHitPoints - nCurrentHitPoints);
ApplyEffectOnObject( DURATION_TYPE_INSTANT, oUser, eHeal );
}
}
// Mark entire map as explored
void main()
{
ExploreAreaForPlayer(OBJECT_SELF,GetEnteringObject());
}
|
|